【symfony2】参考リンク集 & 学習ノート
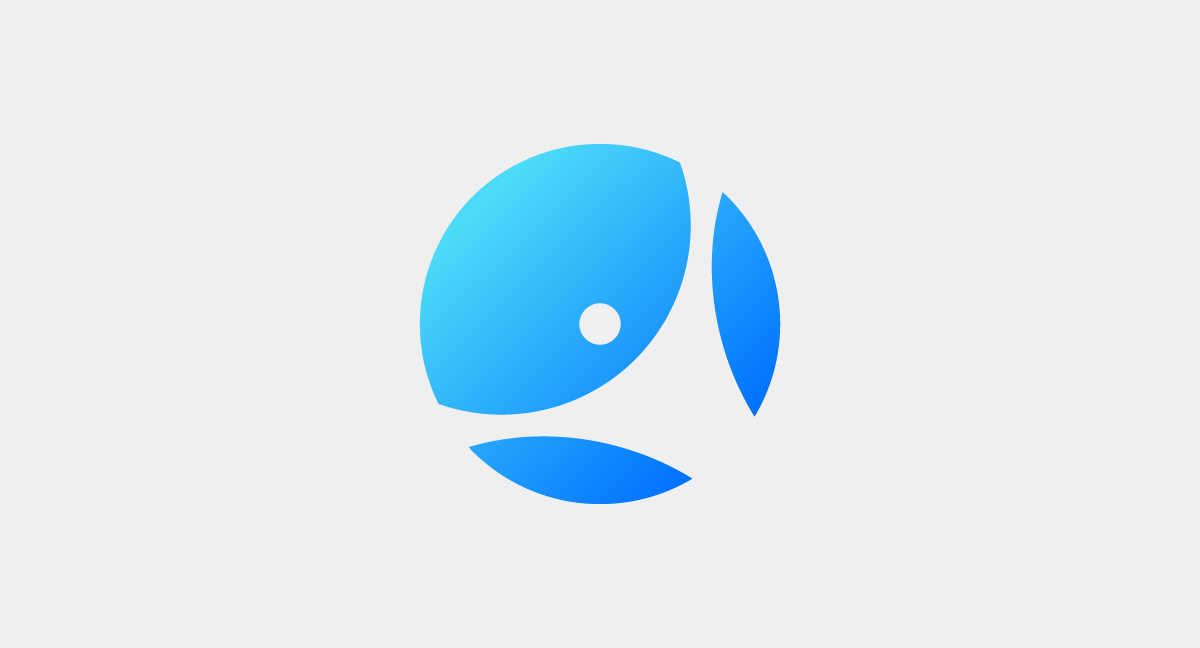
【symfony2】参考リンク集 & 学習ノート
●リンク集
// symfony1との違い
http://docs.symfony.gr.jp/symfony2/cookbook/symfony1.html
// bundles(plugin)を探すなら
http://knpbundles.com/
// namespace
http://d.hatena.ne.jp/hnw/20101006
http://pear.php.net/manual/ja/standards.naming.php
http://php.net/manual/ja/language.namespaces.importing.php// php5.3でuseの使い方
// autoloader
http://docs.symfony.gr.jp/symfony2/cookbook/tools/autoloader.html
// コーディング規約
http://docs.symfony.gr.jp/symfony2/contributing/code/standards.html
http://docs.symfony.gr.jp/symfony2/contributing/documentation/format.html
// doctrine
http://docs.doctrine-project.org/projects/doctrine-orm/en/2.0.x/reference/dql-doctrine-query-language.html
http://docs.doctrine-project.org/projects/doctrine-orm/en/2.0.x/reference/query-builder.html
http://docs.doctrine-project.org/projects/doctrine-orm/en/2.0.x/reference/association-mapping.html
http://docs.doctrine-project.org/projects/doctrine-orm/en/2.0.x/reference/events.html
http://docs.doctrine-project.org/projects/doctrine-orm/en/2.0.x/reference/basic-mapping.html
http://symfony.com/doc/2.0/reference/configuration/doctrine.html
http://docs.symfony.gr.jp/symfony2/cookbook/doctrine/dbal.html
http://d.hatena.ne.jp/taka512/20110727/1311771377// 10分ぐらいで学べるSymfony2 ~Doctrineテーブル作成編~
// twig
http://twig.sensiolabs.org/documentation
http://symfony.com/doc/current/book/templating.html
http://twig.sensiolabs.org/doc/tags/index.html
http://twig.sensiolabs.org/doc/extensions.html
// standloneなコンポーネントの使い方
/jeff/blog/id/?did=20120307173019jeff
// annotationの書き方
// 下記五つの書き方がある
// @Route()
// @Method()
// @ParamConverter()
// @Template()
// @Cache()
http://symfony.com/doc/current/bundles/SensioFrameworkExtraBundle/index.html
// JMSSecurityExtraBundleを使うと、下記なannotationが使えるようになる
// @Secure()
// @SecureParam()
// @SecureReturn()
// @RunAs()
// @SatisfiesParentSecurityPolicy()
http://symfony.com/doc/current/bundles/JMSSecurityExtraBundle/index.html
// テスト
http://docs.symfony.gr.jp/symfony2/book/testing.html
// validation
http://docs.symfony.gr.jp/symfony2/reference/constraints.html
// form
http://docs.symfony.gr.jp/symfony2/book/forms.html#book-forms-type-reference
http://symfony.com/doc/current/reference/forms/twig_reference.html
// security
http://symfony.com/doc/2.0/reference/configuration/security.html
// cache
http://tomayko.com/writings/things-caches-do
http://www.mnot.net/cache_docs/
http://docs.symfony.gr.jp/symfony2/cookbook/cache/varnish.html
http://tools.ietf.org/html/rfc2616
http://tools.ietf.org/wg/httpbis/
// DIコンテナ
http://fabien.potencier.org/article/11/what-is-dependency-injection
http://d.hatena.ne.jp/chobi_e/20101102/1288677784
// 勉強会
http://manjiro.net/symfony/418_symfony2_study_4
// twigとほかのtemplate engineerの比較
http://fabien.potencier.org/article/34/templating-engines-in-php
http://fabien.potencier.org/article/35/templating-engines-in-php-follow-up
// ドキュメント集
http://docs.symfony.gr.jp/
// mongoDB
// bundle=/bundles/DoctrineMongoDBBundle/index
http://www.mongodb.org/
// git
http://docs.symfony.gr.jp/symfony2/cookbook/workflow/new_project_git.html
●学習ノート
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 | // doctrine // データの取得 $repository = $this->getDoctrine()->getRepository('AcmeStoreBundle:Product');// AcmeStoreBundleはshortcut $product = $repository->findBy( array('name' => 'foo'), array('price' => 'ASC') );// order by price asc // データの更新 $em = $this->getDoctrine()->getEntityManager();// entity managerを取得 $em->persist($product);// $productをentity managerの監視の下に $em->flush();// $productの更新があれば、DBも更新する // データの削除 $em->remove($product); $em->flush(); // dqlを使う(手動発行) $em = $this->getDoctrine()->getEntityManager(); $query = $em->createQuery( 'SELECT p FROM AcmeStoreBundle:Product p WHERE p.price > :price ORDER BY p.price ASC' )->setParameter('price', '19.99'); $products = $query->getResult();// データを複数件取得 $product = $query->getSingleResult();// データを一件取得、NoResultException→データがないときのExceptionクラス、NonUniqueResultException→データが複数あるときのExceptionクラス // dqlを使う(query builderを使う) $query = $repository->createQueryBuilder('p') ->where('p.price > :price') ->setParameter('price', '19.99') ->orderBy('p.price', 'ASC') ->getQuery(); // Controller // redirectする use Symfony\Component\HttpFoundation\RedirectResponse; return new RedirectResponse($this->generateUrl('homepage')); // forwordする $httpKernel = $this->container->get('http_kernel'); $response = $httpKernel->forward('AcmeHelloBundle:Hello:fancy', array( 'name' => $name, 'color' => 'green', )); // templateを使う $templating = $this->container->get('templating'); $templating->render('AcmeHelloBundle:Hello:index.html.twig', array('name' => $name)); // symfony2で登録したサービスを使う $request = $this->getRequest(); $response = $this->get('response'); $templating = $this->get('templating'); $router = $this->get('router'); $mailer = $this->get('mailer'); $validator = $this->get('validator'); // 異常処理 throw $this->createNotFoundException('The product does not exist'); throw new \Exception('Something went wrong!'); // ステータスコード 200(デフォルト)の Response を作成 $response = new Response('Hello '.$name, 200); // ステータスコード 200 の JSON レスポンスをを作成 $response = new Response(json_encode(array('name' => $name))); $response->headers->set('Content-Type', 'application/json'); // パラメーター取得 $request = $this->getRequest(); $request->isXmlHttpRequest(); // Ajax リクエストかどうか $request->query->get('page'); // $_GET パラメータを取得 $request->request->get('page'); // $_POST パラメータを取得 // twig // 継承 {% extends 'AcmeArticleBundle::layout.html.twig' %} {% block body %} <h1>Recent Articles<h1> {% for article in articles %} {% include 'AcmeArticleBundle:Article:articleDetails.html.twig' with {'article': article} %} {% endfor %} {% endblock %} // コントローラを埋め込む→コントローラの書き方? <div id="sidebar"> {% render "AcmeArticleBundle:Article:recentArticles" with {'max': 3} %} </div> // 相対URL <a href="{{ path('_welcome') }}">Home</a> // 絶対URL <a href="{{ url('_welcome') }}">Home</a> // asset <img src="{{ asset('images/logo.png') }}" alt="Symfony!" /> // css {% block stylesheets %} {{ parent() }} <link href="{{ asset('/css/contact.css') }}" type="text/css" rel="stylesheet" /> {% endblock %} // javascriptファイルをひとつファイルにまとめて出力 {% javascripts '@AcmeFooBundle/Resources/public/js/*' '@AcmeBarBundle/Resources/public/js/form.js' '@AcmeBarBundle/Resources/public/js/calendar.js' %} <script src="{{ asset_url }}"></script> {% endjavascripts %} // JSフィルタの設定 # app/config/config.yml assetic: filters: yui_js: jar: "%kernel.root_dir%/Resources/java/yuicompressor.jar" // コアテンプレートをオーバーライドする、下記ファイルを作る app/Resources/TwigBundle/views/Exception // TwigBundleのExceptionをオーバーライドしたい場合 // テープレート3level継承 1、app/Resources/views/base.html.twig 2、AcmeBlogBundle::layout.html.twig 3、AcmeBlogBundle:Blog:index.html.twig // アウトプットエスケープ off {{ article.body | raw }} // requestのファイルフォーマットを取得 $format = $this->getRequest()->getRequestFormat(); // form // action側 $product = new Product(); $form = $this->createFormBuilder($product) ->add('name', 'text') ->add('price', 'money', array('currency' => 'USD')) ->getForm(); // viewだけ作るなら return $this->render('AcmeStoreBundle:Default:index.html.twig', array( 'form' => $form->createView(), )); // bindするなら if ($request->getMethod() == 'POST') { $form->bindRequest($request); if ($form->isValid()) { // データベースへの保存など、何らかのアクションを実行する return $this->redirect($this->generateUrl('store_product_success')); } } // template側 // form全体を出力 <form action="{{ path('store_product') }}" method="post" {{ form_enctype(form) }}> {{ form_widget(form) }} <input type="submit" /> </form> // formを個別項目で出力 <form action="{{ path('store_product') }}" method="post" {{ form_enctype(form) }}> {{ form_errors(form) }} {{ form_row(form.name) }} {{ form_row(form.price) }} {{ form_rest(form) }} <input type="submit" /> </form> // form_rowのカスタマイズ <div> {{ form_label(form.name) }} {{ form_errors(form.name) }} {{ form_widget(form.name) }} </div> |
●おまけ symfony2でのconsoloの使い方
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | // doctrine php app/console doctrine:database:create php app/console doctrine:generate:entity --entity="AcmeStoreBundle:Product" --fields="name:string(255) price:float description:text" // --format=ymlのオプションを追加すれば、metadataとして、ymlファイルが作成される php app/console doctrine:schema:update --force php app/console doctrine:schema:create // システムのサービスリストを調べる php app/console container:debug // routing設定を表示する php app/console router:debug // asset // アセットファイルをネットから参照する場合、ローカルにコーピおよびシンボリックリンクを作る php app/console assets:install target [--symlink] // アセットファイルの更新有無をチェック php app/console assetic:dump |
Author Profile
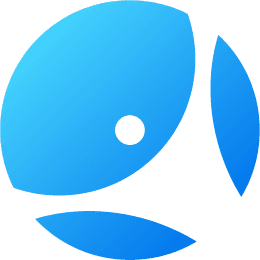
スターフィールド編集部
SHARE