cakephp 一覧表示、登録、編集、削除の実装
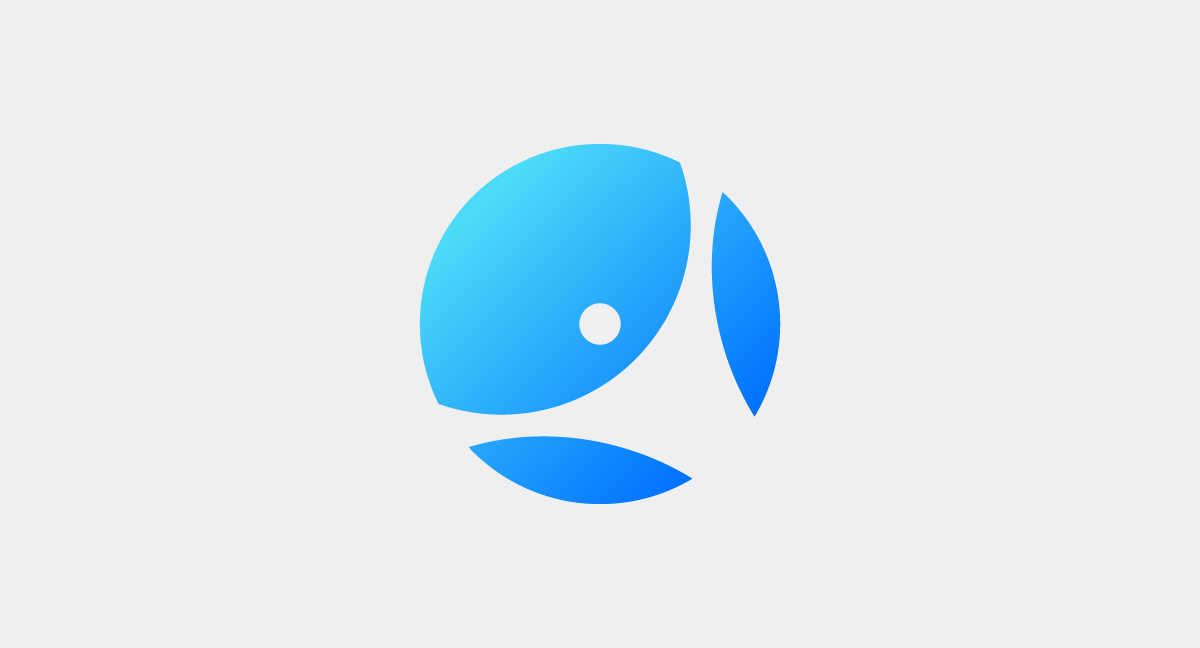
cakephpで登録処理(一覧表示、登録、編集、削除)の一連の実装をやっていきます。
一覧表示
予め、DBにテーブルを作っておきます。
今回は簡単な名前、年齢などが格納されたテーブルを作成します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | // テーブル作成 CREATE TABLE IF NOT EXISTS `samples` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(100) NOT NULL, `age` tinyint(4) NOT NULL, `created` datetime NOT NULL, `modified` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ; // データインサート INSERT INTO `samples` (`id`, `name`, `age`, `created`, `modified`) VALUES (1, '佐藤', 25, '2015-04-07 00:56:23', '2015-04-07 00:56:23'), (2, '中山', 23, '2015-04-07 01:45:19', '2015-04-07 01:46:15'), (3, '宮田', 27, '2015-04-07 01:46:32', '2015-04-07 01:46:32'); |
・Modelの作成
モデルはデータベースのテーブルと繋がるもので基本的にテーブル名と同じ+頭文字を大文字+単数形でつくります。今回はsamplesというテーブルなのでモデル名はSample.phpとなります。これをModelフォルダに作成し、Modelファイルを作成します。
例:app/Model/Sample.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | <?php class Sample extends AppModel{ public $name = 'Sample'; public $validate = array( 'name' => array( 'rule' => array('between',0,10), 'required' => true, 'alloEmpty' => false, 'message' => '10文字以内で必ず入力して下さい' ), 'age' => array( 'rule' => array('custom','/^[0-9]{1,3}$/'), 'required' => true, 'alloEmpty' => false, 'message' => '数字3桁以内で必ず入力して下さい' ), ); } |
・コントローラーの作成
コントローラーを作成してデータを取得します。
コントローラーはモデル名+複数形+Controllerでファイル名を作成します。ここではSamplesController.phpとなります。このファイルをControllerフォルダに作成します。
例:app/Controller/SamplesController.php
1 2 3 4 5 6 7 8 9 10 11 | <?php class SamplesController extends AppController { public $name = 'Samples'; public $uses = array("Sample"); public function lists() { // set('送信する変数名',$this->Model名->find('条件')); $data = $this->Sample->find('all'); $this->set('data', $data); } } |
・ビューの作成
コントローラーを取得したデータをビューで表示させます。
Viewにコントローラーと同名のフォルダを作成、その中にアクション(メソッド)名と同じテンプレートを作成となります。
例:app/View/Samples/lists.ctp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | <table style="margin-bottom: 45px;"> <tr> <th>お名前</th> <th>年齢</th> <th>登録日時</th> <th>編集</th> <th>削除</th> </tr> <?php foreach ($data as $contact): ?> <tr> <td><?php echo $contact['Sample']['name']; ?></td> <td><?php echo $contact['Sample']['age']; ?></td> <td><?php echo $contact['Sample']['created']; ?></td> <td><?php echo $this->Html->link('編集',array('action'=>'edit',$contact['Sample']['id'])); ?></td> <td><?php echo $this->Html->link('削除',array('action'=>'delete',$contact['Sample']['id'])); ?></td> </tr> <?php endforeach; ?> </table> <?php echo $this->Html->link('追加',array('action'=>'add')); ?> |
一覧表示に必要な設定は終わったのでアクセスしてみます。
一覧表示
これで一覧表示は完成です。
登録
新規登録ページを作成します。
コントローラーに新規登録のメソッドを追加します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class SamplesController extends AppController { public $name = 'Samples'; public $uses = array("Sample"); public function lists() { // set('送信する変数名',$this->Model名->find('条件')); $data = $this->Sample->find('all'); $this->set('data', $data); } public function add() { if($this->request->is('post')) { if($this->Sample->save($this->request->data)) { $this->Session->setFlash('入力完了'); $this->redirect(array('action'=>'lists')); } else { $this->Session->setFlash('入力失敗'); } } } } |
これで新規登録ができるようになったので、登録画面にアクセスします。
登録画面
編集
登録と同様に編集のメソッドをコントローラーに追加します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | class SamplesController extends AppController { public $name = 'Samples'; public $uses = array("Sample"); public function lists() { // set('送信する変数名',$this->Model名->find('条件')); $data = $this->Sample->find('all'); $this->set('data', $data); } public function add() { if($this->request->is('post')) { if($this->Sample->save($this->request->data)) { $this->Session->setFlash('入力完了'); $this->redirect(array('action'=>'lists')); } else{ $this->Session->setFlash('入力失敗'); } } } public function edit($id = null) { $this->Sample->id = $id; if($this->request->is('get')) { $this->request->data=$this->Sample->read(); //更新画面の表示 } else { if($this->Sample->save($this->request->data)) { $this->Session->setFlash('更新完了'); $this->redirect(array('action'=>'lists')); } else { $this->Sessin->setFlash('更新失敗'); } } } } |
これで編集ができるようになったので、一覧画面にアクセスして
編集ボタンをクリックします。
一覧画面
削除
編集と同様、各データごとに削除ボタンを設置します。
削除のメソッドをコントローラーに追加します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | <?php class SamplesController extends AppController { public $name = 'Samples'; public $uses = array("Sample"); public function lists() { $data = $this->Sample->find('all'); $this->set('data', $data); } public function add() { if($this->request->is('post')) { if($this->Sample->save($this->request->data)) { $this->Session->setFlash('入力完了'); $this->redirect(array('action'=>'lists')); } else { $this->Session->setFlash('入力失敗'); } } } public function edit($id = null) { $this->Sample->id = $id; if($this->request->is('get')) { $this->request->data=$this->Sample->read(); } else { if($this->Sample->save($this->request->data)) { $this->Session->setFlash('更新完了'); $this->redirect(array('action'=>'lists')); } else { $this->Sessin->setFlash('更新失敗'); } } } public function delete($id=null) { $this->Sample->id = $id; if($this->Sample->delete()){ $this->Session->setFlash('削除完了'); $this->redirect(array('action'=>'lists')); } else { $this->Sessin->setFlash('削除失敗'); } } } |
これで削除ができるようになったので、一覧画面にアクセスして
削除ボタンをクリックします。
一覧画面
以上になります。
Author Profile
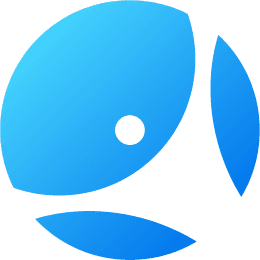
スターフィールド編集部
SHARE